Aggressive Development
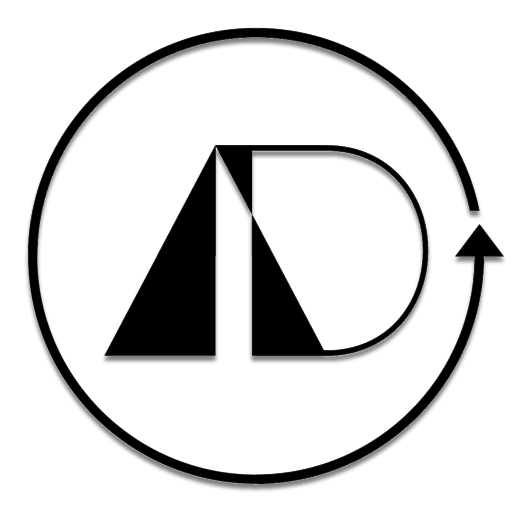
A company developing apps and websites since 2009. Based in Sweden, crafting delicious apps furiously. Currently not as aggressively as it used to. Aggressive in moderation.
Aggressive Development has published 13 apps under its own name, and many more for other companies. More than 60 magazine-apps have been built for Swedish publishers, and here you can find those who are still active: Qiozk Solo Magazines.
I am currently working as a senior mobile developer at Qvik. Here is My resume
Feeds is a news/rss app that streams webpages into your pocket. Feeds is the next application from the furious mind of Aggressive Development (that's me) - giving everyone easy access to news and blogs in the most elegant and efficient way possible. Everything streamlined in a convenient feed.
Sounds interesting? Read more about Feeds: